springMVC + iBatis 테스트 정리
환경 : NT, eclipse, mysql, Tomcat6.0
File -> new -> Dynamic web project -> Sample (프로젝트명)
-------------------------------------------------------------------------------------------
먼저 web.xml 아래와 같이 작성
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID" version="2.5">
<display-name>Sample</display-name>
<welcome-file-list>
<welcome-file>/WEB-INF/jsp/redirect.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>
org.springframework.web.filter.CharacterEncodingFilter
</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
<context-param> <!-- log4j 사용 -->
<param-name>log4jConfigLocation</param-name>
<param-value>/WEB-INF/properties/log4j.properties</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.util.Log4jConfigListener</listener-class>
</listener>
</web-app>
-------------------------------------------------------------------------------------------
WEB-INF/dispatcher-servlet.xml 생성
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation=" http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- HandlerMapping -->
<bean id="sampleMapping" class="org.springframework.web.servlet.handler.BeanNameUrlHandlerMapping">
</bean>
<!-- Controller -->
<bean id="sampleController" name="/index.do" class="com.younghoi.sample.controller.SampleController">
<property name="sampleService" ref="sampleService"/>
</bean>
<!-- service -->
<bean id="sampleService" class="com.younghoi.sample.service.SampleService">
<property name="sampleDao" ref="sampleDao"/>
</bean>
<!-- Data Source -->
<bean id="dataSource" class="org.springframework.jndi.JndiObjectFactoryBean">
<property name="jndiName" value="java:/comp/env/sampledb" />
</bean>
<!-- Transaction Manager for a single JDBC DataSource -->
<bean id="txManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
<!-- SqlMap setup for iBATIS Database Layer -->
<bean id="sqlMapClient" class="org.springframework.orm.ibatis.SqlMapClientFactoryBean">
<property name="configLocation" value="/WEB-INF/sqlMapConfig.xml"/>
<property name="dataSource" ref="dataSource"/>
</bean>
<!-- sampleDao -->
<bean id="sampleDao" class="com.younghoi.sample.dao.SampleDao" autowire="byName">
<property name="sqlMapClient" ref="sqlMapClient"/>
</bean>
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix">
<value>/WEB-INF/jsp/</value>
</property>
<property name="suffix">
<value>.jsp</value>
</property>
</bean>
</beans>
-------------------------------------------------------------------------------------------
WEB-INF/sqlMapConfig.xml 생성
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE sqlMapConfig PUBLIC "-//iBATIS.com//DTD SQL Map Config 2.0//EN" "WEB-INF/sql-map-config-2.dtd">
<sqlMapConfig>
<settings cacheModelsEnabled="false" useStatementNamespaces="true"/>
<sqlMap resource="com/younghoi/sample/dao/map/sample.xml"/>
</sqlMapConfig>
-------------------------------------------------------------------------------------------
WEB-INF/properties/log4j.properties 위치 시킴
-------------------------------------------------------------------------------------------
src/com/younghoi/sample/dto/Item.java 생성
package com.younghoi.sample.dto;
public class Item {
private Integer itemId;
private String itemName;
private Integer price;
private String description;
private String pictureUrl;
public Integer getItemId() {
return itemId;
}
public void setItemId(Integer itemId) {
this.itemId = itemId;
}
public String getItemName() {
return itemName;
}
public void setItemName(String itemName) {
this.itemName = itemName;
}
public Integer getPrice() {
return price;
}
public void setPrice(Integer price) {
this.price = price;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getPictureUrl() {
return pictureUrl;
}
public void setPictureUrl(String pictureUrl) {
this.pictureUrl = pictureUrl;
}
}
-------------------------------------------------------------------------------------------
src/com/younghoi/sample/controller/SampleController.java 생성
package com.younghoi.sample.controller;
import java.util.*;
import javax.servlet.http.*;
import org.springframework.web.servlet.*;
import org.springframework.web.servlet.mvc.*;
import com.younghoi.sample.dto.*;
import com.younghoi.sample.service.*;
/**
* @file SampleController.java
* @description : sample controller class
* @author young-hoi.kim
* @mail young-hoi.kim@livestep.co.kr
* @since 2011. 10. 13.
*/
public class SampleController implements Controller{
private SampleServiceI sampleServiceI;
public void setSampleService(SampleServiceI sampleServiceI){
this.sampleServiceI = sampleServiceI;
}
@Override
public ModelAndView handleRequest(HttpServletRequest arg0,HttpServletResponse arg1) throws Exception {
List<Item> itemList = this.sampleServiceI.getItemList();
ModelAndView mav = new ModelAndView();
mav.addObject(itemList);
mav.setViewName("item");
return mav;
}
}
-------------------------------------------------------------------------------------------
src/com/younghoi/sample/service/SampleServiceI.java (interface) 생성
package com.younghoi.sample.service;
import java.util.*;
import org.springframework.dao.*;
import com.younghoi.sample.dto.*;
/**
* @file SampleServiceI.java
* @description : sample service interface
* @author young-hoi.kim
* @mail spdlqjdudghl@naver.com
* @since 2011. 10. 13.
*/
public interface SampleServiceI {
/**
* @description : item 정보 list 가져오기
* @author young-hoi.kim
* @since 2011. 10. 13.
* @return List<Item>
*/
public List<Item> getItemList() throws DataAccessException, Exception;
}
-------------------------------------------------------------------------------------------
src/com/younghoi/sample/service/SampleService.java 생성
-------------------------------------------------------------------------------------------
src/com/younghoi/sample/dao/SampleDaoI.java (interface) 생성
package com.younghoi.sample.dao;
import java.util.*;
import org.springframework.dao.*;
import com.younghoi.sample.dto.*;
/**
* @file SampleDaoI.java
* @description : sample dao interface
* @author young-hoi.kim
* @mail young-hoi.kim@livestep.co.kr
* @since 2011. 10. 13.
*/
public interface SampleDaoI {
/**
* @description : item 정보 list 가져오기
* @author young-hoi.kim
* @since 2011. 10. 13.
* @return List<Item>
*/
public List<Item> getItemList() throws DataAccessException, Exception;
}
-------------------------------------------------------------------------------------------
src/com/younghoi/sample/dao/SampleDao.java 생성
package com.younghoi.sample.dao;
import java.util.*;
import org.springframework.dao.*;
import org.springframework.orm.ibatis.support.*;
import com.younghoi.sample.dto.*;
/**
* @file SampleDao.java
* @description : sample dao implements class
* @author young-hoi.kim
* @mail young-hoi.kim@livestep.co.kr
* @since 2011. 10. 13.
*/
public class SampleDao extends SqlMapClientDaoSupport implements SampleDaoI{
@SuppressWarnings("unchecked")
@Override
public List<Item> getItemList() throws DataAccessException, Exception {
List<Item> rtnList = null;
try{
rtnList = getSqlMapClientTemplate().queryForList("sample.getItemList");
}catch(DataAccessException e){
logger.error("SampleDao DataAccess error : getItemList ==>",e);
throw e;
}catch(Exception e){
logger.error("SampleDao error : getItemList ==>",e);
throw e;
}
return rtnList;
}
}
-------------------------------------------------------------------------------------------
src/com/younghoi/sample/dao/map/sample.xml 생성
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE sqlMap
PUBLIC "-//ibatis.apache.org//DTD SQL Map 2.0//EN"
"http://ibatis.apache.org/dtd/sql-map-2.dtd">
<sqlMap namespace="sample">
<typeAlias alias="Item" type="com.younghoi.sample.dto.Item"/>
<resultMap id="itemList" class="Item">
<result property="itemId" column="itemid"/>
<result property="itemName" column="itemname"/>
<result property="price" column="price"/>
<result property="description" column="description"/>
<result property="pictureUrl" column="pictureurl"/>
</resultMap>
<!-- item 정보 리스트 조회 -->
<select id="getItemList" resultMap="itemList">
SELECT itemid, itemname, price, description, pictureurl FROM item
</select>
</sqlMap>
-------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------
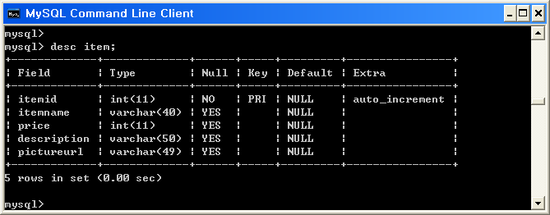
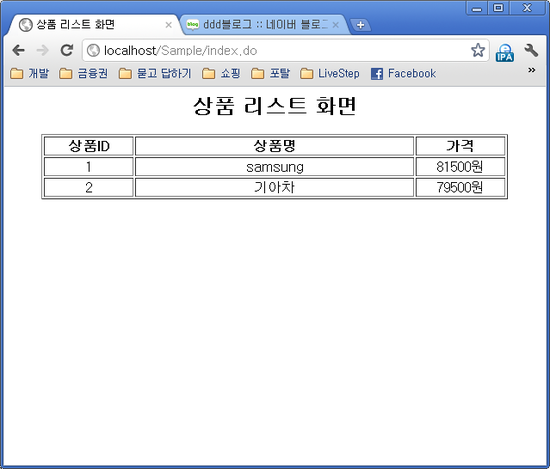
'IT > Spring' 카테고리의 다른 글
ehcache (0) | 2013.12.24 |
---|---|
spring file download (0) | 2013.12.24 |
Spring2.5 Ajax (0) | 2013.12.24 |
udp-inbound-channel-adapter 이용 was 에 udp server socket 구성 (0) | 2013.12.24 |
spring + tiles (0) | 2013.12.24 |